It’s really useful to have a simple way of seeing what code you have deployed.
Any Spring Boot application can add a simple git info endpoint using out-of-the-box actuator functionality and a simple maven plugin.
This allows you to do a simple curl on a URL and see exactly what version of your code is running by exposing the git info.
Step 1 – Create a Simple Spring Boot Application
The following command will use the spring initializer project to create a new spring boot application and place it in the /springboot directory.
curl -G https://start.spring.io/starter.tgz -d dependencies=web,actuator -d baseDir=springboot -d groupId=com.sdbc | tar -xzvf -
Step 2 – Create your git repo
Run the following command to set up your git repo
cd springboot
git init
git add --all
git commit -m "initial code"
git remote add origin ./.git # keep it all local
This will look as follows.
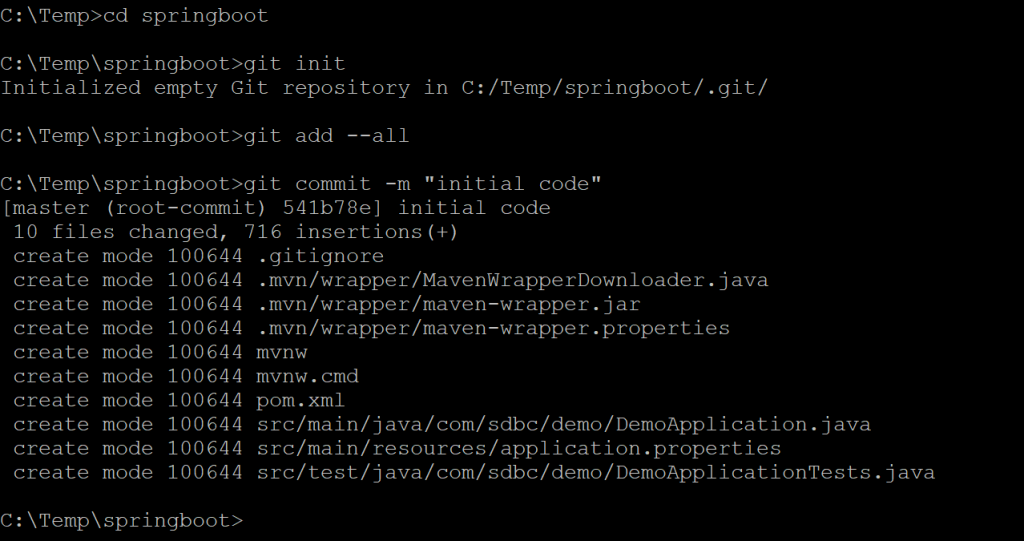
This enables the plugin to pull back the git info for the project.
Step 3 – Add the Maven plugin
Add the following plugin to your pom.xml.
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>pl.project13.maven</groupId>
<artifactId>git-commit-id-plugin</artifactId>
</plugin>
</plugins>
</build>
The plugin will automatically add the build information to a file git.properties in the target/classes folder when you build your code.
Run the following command and then check the properties.
mvn clean install
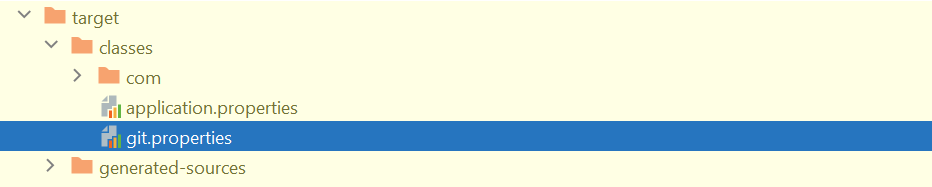
This contains all of the following information.

For full details on the plugin see the references below.
Step 4 – Expose the information.
By default, a Spring Boot application hides the info actuator endpoint. We generally change this in properties. But for this blog, we will just pass it as a property when running the application.
Start the application as below.
mvn spring-boot:run -Dspring-boot.run.arguments=
--management.endpoints.web.exposure.include=info
You can then hit the endpoint in the browser.
http://localhost:8080/actuator/info
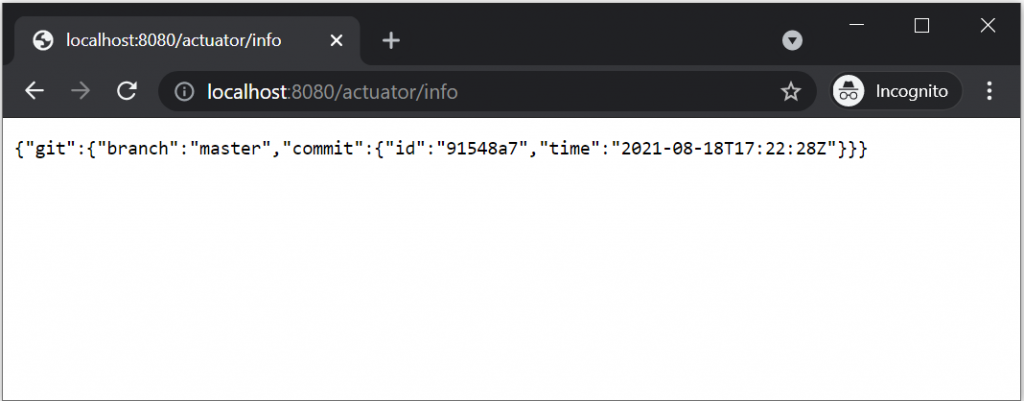
Check the reference on the plugin to see how you can customise this information.
References
https://docs.spring.io/spring-boot/docs/current/reference/html/actuator.html
https://github.com/git-commit-id/git-commit-id-maven-plugin
Leave a Reply
You must be logged in to post a comment.