At some point, you are going to have to stub your services [1]. This is a quick example of how you can serve up JSON by running a standalone stubbing service with WireMock[2]
WireMock is often used in Java projects but is completely standalone.
Running The Example.
Let’s run the final code before we explain how it works.
Clone the code and then run the local server as follows.
$ git clone git@github.com:johndobie-blog/wiremock.git
$ cd wiremock/src
$ java -cp "slf4j-nop-1.7.9.jar;wiremock-standalone-2.25.1.jar" com.github.tomakehurst.wiremock.standalone.WireMockServerRunner --port 9999 --verbose
You can then hit the following URL to see the stubbed JSON data.
http://localhost:9999/details
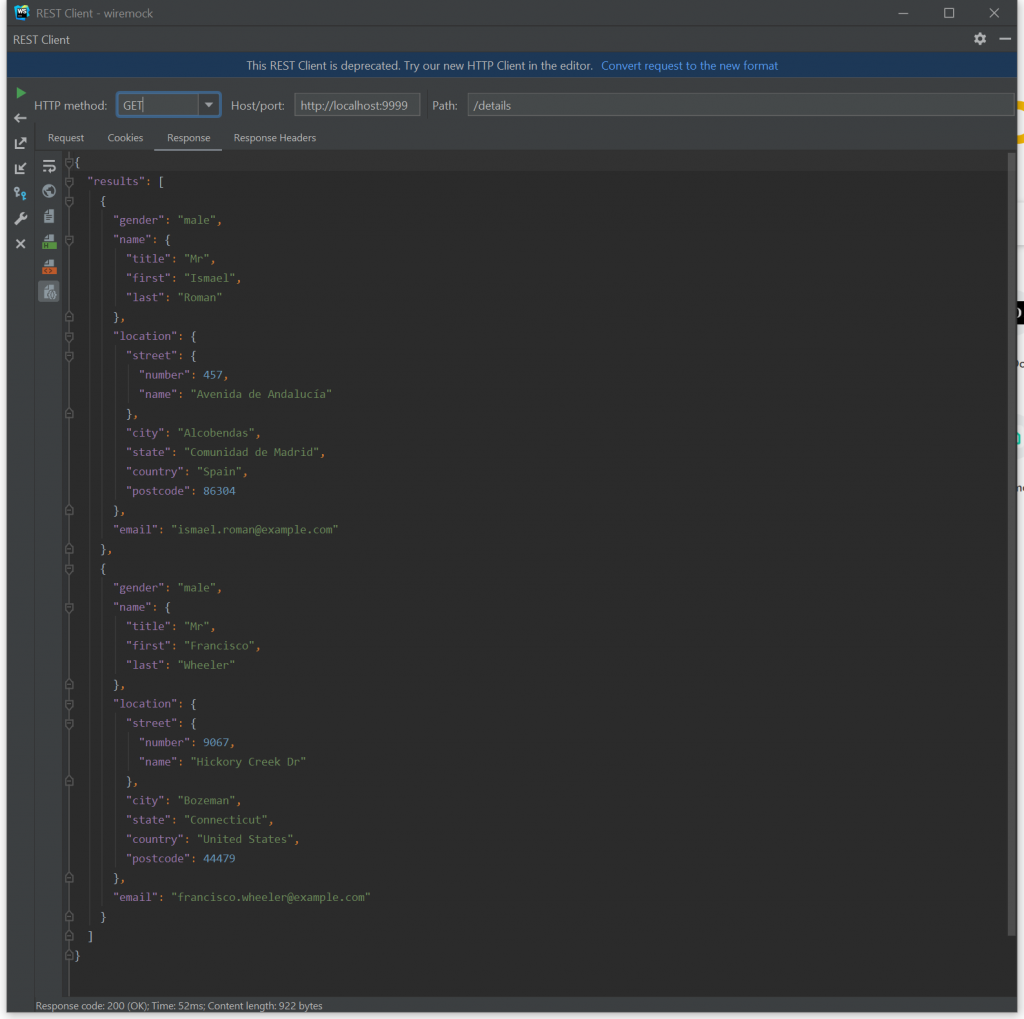
Setting Up WireMock
Setting up WireMock is very simple. You need the following 2 jars.
The second jar is required if you want to see any meaningful logging.
$ slf4j-nop-1.7.9.jar wiremock-standalone-2.25.1.jar
You can then start the WireMock server on port 9999 as follows.
$ java -cp "slf4j-nop-1.7.9.jar;wiremock-standalone-2.25.1.jar" com.github.tomakehurst.wiremock.standalone.WireMockServerRunner --port 9999 --verbose
At this point, it will not serve up any data. For that, you will need to add some stub files and some mappings.
Creating The Stubs
The stubs are created in the src__files\files directory
For our example, we are going to create a file called details.json which will contain the above served up data.
{
"results": [
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Ismael",
"last": "Roman"
},
"location": {
"street": {
"number": 457,
"name": "Avenida de Andalucía"
},
"city": "Alcobendas",
"state": "Comunidad de Madrid",
"country": "Spain",
"postcode": 86304
},
"email": "ismael.roman@example.com"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Francisco",
"last": "Wheeler"
},
"location": {
"street": {
"number": 9067,
"name": "Hickory Creek Dr"
},
"city": "Bozeman",
"state": "Connecticut",
"country": "United States",
"postcode": 44479
},
"email": "francisco.wheeler@example.com"
}
]
}
Creating The Mappings
The mapping is very simple.
{
"request": {
"method": "GET",
"url": "/details"
},
"response": {
"status": 200,
"bodyFileName" : "files/details.json",
"headers": {
"Content-Type": "application/json",
"Access-Control-Allow-Origin" : "*",
"Access-Control-Allow-Methods" : "*",
"Access-Control-Allow-Headers": "Accept, Content-Type, Content-Encoding, Server, Transfer-Encoding"
}
}
}
Most of the attributes should be self-explanatory, except perhaps for the headers. You can ignore the details but they are required for local cross-origin requests.
Basically we have defined a path /details which will serve up the JSON that we defined in our details.json file.
Viewing In The Browser
Simply point your browser at the url http://localhost:9999/details
Alternatively, you can use the following curl command.
$ curl http://localhost:9999/details
{
"results": [
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Ismael",
"last": "Roman"
},
"location": {
"street": {
"number": 457,
"name": "Avenida de Andalucía"
},
"city": "Alcobendas",
"state": "Comunidad de Madrid",
"country": "Spain",
"postcode": 86304
},
"email": "ismael.roman@example.com"
},
{
"gender": "male",
"name": {
"title": "Mr",
"first": "Francisco",
"last": "Wheeler"
},
"location": {
"street": {
"number": 9067,
"name": "Hickory Creek Dr"
},
"city": "Bozeman",
"state": "Connecticut",
"country": "United States",
"postcode": 44479
},
"email": "francisco.wheeler@example.com"
}
]
}
References
[1] Test Doubles With Mockito.
[2] WireMock. https://wiremock.com
Leave a Reply
You must be logged in to post a comment.